Expression Gallery
With the Node Expressions add-on, paste these expressions into a new text block in the text editor and give it suitable name. Then simply add a new expression of 'TEXT:<name>' (where <name> is the name of the text block) to create the node group for the following examples and simply hook up the nodes as shown in the example usage.

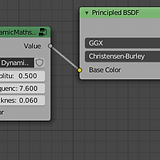
Wave
Beginner
Value = abs(Vector[x]-(sin(Vector[y]*Frequency{10})*Amplitude{0.9}))>Thickness{0.1}
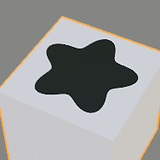

Star
Intermediate
_x = Vector[x] , _y = Vector[y]
_dist=(_x**2+_y**2)**0.5
_angle=atan2(_y,_x)
_edge=sin(_angle*Points{5})/2+0.5
Output=_dist>_edge*(Outer{0.45}-Inner{0.25})+Inner


Polygon
Intermediate
_x = Input[x]
_y = Input[y]
_dist = (_x*_x + _y*_y) **0.5
_angle = atan2(_x,_y) / 3.1415 / 2 + 0.5
_edge = round(_angle * Sides)/Sides
_edgeAngle = _angle - _edge
Edge = (Radius / cos(_edgeAngle * 3.1415 * 2*Pointiness{1})) - _dist
Output = Edge > 0

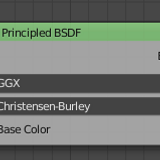
Hexagons
Advanced
_x=Input.Generated[x], _y=Input.Generated[y]
#Set X and Y scaling based on Aspect ratio (0.866 for undistorted hexagons (`sin(60 degrees)`)
_xpitch=Aspect{1.0}/Scale{5}
_ypitch=0.866/Scale
#Logic to control where we need to place the reference points
_firsthalf=mmod(_x,_xpitch)>_xpitch/2
_tophalf=mmod(_y,_ypitch)>_ypitch/2
_oddline=mmod(_y,_ypitch*2)>_ypitch
#Define the reference points - x1 is "main" reference, others positioned in relation to that
_x1=_x-mmod(_x,_xpitch)+_oddline*((-_xpitch/2*0)), _y1=_y-mmod(_y,_ypitch)+_oddline*_ypitch
_x2=_x1+_xpitch, _y2=_y1
_x3=(_x1+_x2)/2, _y3=_y1-_ypitch
_x4=_x3, _y4=_y1+_ypitch
_x5=_x3-_xpitch+_xpitch*2*_firsthalf, _y5=_y3*not(_tophalf)+_y4*_tophalf
#Distance to each reference point
_d1 = ((_x-_x1)**2+(_y-_y1)**2)**0.5
_d2 = ((_x-_x2)**2+(_y-_y2)**2)**0.5
_d3 = ((_x-_x3)**2+(_y-_y3)**2)**0.5
_d4 = ((_x-_x4)**2+(_y-_y4)**2)**0.5
_d5 = ((_x-_x5)**2+(_y-_y5)**2)**0.5
#Distance to the closest point
_dist = min(_d1,_d2,_d3,_d4,_d5)
#Determine which of the reference point is the closest
_closest1 = (_dist == _d1)
_closest2 = not(_closest1)*(_dist == _d2)
_closest3 = not(or(_closest1,_closest2))*(_dist == _d3)
_closest4 = not(or(_closest1,_closest2,_closest3))*(_dist == _d4)
_closest5 = not(or(_closest1,_closest2,_closest3,_closest4))
#Determine the distance to the *next* closest
_nextdist = max(_closest1*min(_d2, _d3, _d4, _d5)
_closest2*min(_d1, _d3, _d4, _d5)
_closest3*min(_d1, _d2, _d4, _d5)
_closest4*min(_d1, _d2, _d3, _d5)
_closest5*min(_d1, _d2, _d3, _d4, _d5))
#Calculate how close we are to the edge (the edge is halfway between the two distances)
Hexagon = 1-(_dist /((_nextdist + _dist)/2))


Mandelbulb
Advanced
#Define the group for one 'iteration' of the Mandelbulb calculation
group:start(mandelbulbiteration, N, iBlown, Limit, iOffset[], iVector[])
#Split the input vector into x,y,z
_x = iVector[x]
_y = iVector[y]
_z = iVector[z]
#Calculate the coordinates
_r = (_x*_x + _y*_y + _z*_z)**0.5
_theta = atan2((_x*_x+_y*_y)**0.5,_z)
_phi = atan2(_y,_x)
_rn = _r ** N
_newx = _rn * sin(_theta*N) * cos(_phi*N)
_newy = _rn * sin(_theta*N) * sin(_phi*N)
_newz = _rn * cos(_theta*N)
#New distance from centre
_newR = (_newx*_newx + _newy*_newy + _newz*_newz)**0.5
#Determine whether our new point lies outside the sphere
oBlown = or(iBlown, _newR> Limit)
oVector[] = vadd(combine(_newx, _newy, _newz), iOffset[])
Intensity = _r - _newR
#End the group - the name should match the 'start'
group:end(mandelbulbiteration)
#-----------------------------------------------------------------------------------------
#Add a group node named 'iteration1', setting the inputs to the group input values
group:add(mandelbulbiteration,iteration1,N{8},0,Limit{2},Input[], Input[])
#Add second group node named iteration2 and linked to the first
group:add(mandelbulbiteration,iteration2,N,group:iteration1[oBlown],Limit,Input[], group:iteration1[oVector])
#Add some more iterations
group:add(mandelbulbiteration,iteration3,N,group:iteration2[oBlown],Limit,Input[], group:iteration2[oVector])
group:add(mandelbulbiteration,iteration4,N,group:iteration3[oBlown],Limit,Input[], group:iteration3[oVector])
group:add(mandelbulbiteration,iteration5,N,group:iteration4[oBlown],Limit,Input[], group:iteration4[oVector])
group:add(mandelbulbiteration,iteration6,N,group:iteration5[oBlown],Limit,Input[], group:iteration5[oVector])
group:add(mandelbulbiteration,iteration7,N,group:iteration6[oBlown],Limit,Input[], group:iteration6[oVector])
group:add(mandelbulbiteration,iteration8,N,group:iteration7[oBlown],Limit,Input[], group:iteration7[oVector])
group:add(mandelbulbiteration,iteration9,N,group:iteration8[oBlown],Limit,Input[], group:iteration8[oVector])
group:add(mandelbulbiteration,iteration10,N,group:iteration9[oBlown],Limit,Input[], group:iteration9[oVector])
group:add(mandelbulbiteration,iteration11,N,group:iteration10[oBlown],Limit,Input[], group:iteration10[oVector])
group:add(mandelbulbiteration,iteration12,N,group:iteration11[oBlown],Limit,Input[], group:iteration11[oVector])
group:add(mandelbulbiteration,iteration13,N,group:iteration12[oBlown],Limit,Input[], group:iteration12[oVector])
group:add(mandelbulbiteration,iteration14,N,group:iteration13[oBlown],Limit,Input[], group:iteration13[oVector])
group:add(mandelbulbiteration,iteration15,N,group:iteration14[oBlown],Limit,Input[], group:iteration14[oVector])
group:add(mandelbulbiteration,iteration16,N,group:iteration15[oBlown],Limit,Input[], group:iteration15[oVector])
group:add(mandelbulbiteration,iteration17,N,group:iteration16[oBlown],Limit,Input[], group:iteration16[oVector])
group:add(mandelbulbiteration,iteration18,N,group:iteration17[oBlown],Limit,Input[], group:iteration17[oVector])
group:add(mandelbulbiteration,iteration19,N,group:iteration18[oBlown],Limit,Input[], group:iteration18[oVector])
#Define the outputs
Inside = not(group:iteration19[oBlown])
Intensity = group:iteration19[Intensity]